Bài 6 – Lấy dữ liệu có điều kiện từ bảng cơ sở dữ liệu MySQL trong Python
Thông thường chúng ta chỉ lấy các bản ghi trong bảng thỏa mãn điều kiện, do đó ta sẽ đặt điều kiện thông qua câu lệnh WHERE của SQL khi truy vấn.
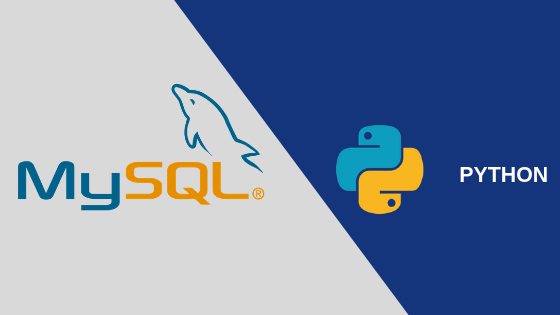
Lọc bản ghi thỏa mãn điều kiện
import mysql.connector
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="mydatabase"
)
mycursor = mydb.cursor()
sql = "SELECT * FROM customers WHERE address ='Park Lane 38'"
mycursor.execute(sql)
myresult = mycursor.fetchall()
for x in myresult:
print(x)
Lọc bản ghi sử dụng ký tự đại diện
Chúng ta sẽ sử dụng ký tự % để đại diện cho các ký tự bất kỳ. Ví dụ lọc các bản ghi thỏa mãn address có chứa chữ way
import mysql.connector
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="mydatabase"
)
mycursor = mydb.cursor()
sql = "SELECT * FROM customers WHERE address LIKE '%way%'"
mycursor.execute(sql)
myresult = mycursor.fetchall()
for x in myresult:
print(x)
Thay thế giá trị khi truy vấn
import mysql.connector
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="mydatabase"
)
mycursor = mydb.cursor()
sql = "SELECT * FROM customers WHERE address = %s"
adr = ("Yellow Garden 2", )
mycursor.execute(sql, adr)
myresult = mycursor.fetchall()
for x in myresult:
print(x)
Lưu ý: Sử dụng %s để đại diện cho các giá trị được thay thế.
Sắp xếp các bản ghi khi truy vấn
Để sắp xếp các bản ghi khi truy vấn ta sử dụng câu lệnh ORDER BY của SQL trong câu lệnh SELECT. Theo mặc định thì sắp xếp tăng dần khi sử dụng ORDER BY, nếu muốn sắp xếp giảm dần thì chúng ta sử dụng từ khóa DESC.
Ví dụ sắp xếp tăng dần của trường name
import mysql.connector
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="mydatabase"
)
mycursor = mydb.cursor()
sql = "SELECT * FROM customers ORDER BY name"
mycursor.execute(sql)
myresult = mycursor.fetchall()
for x in myresult:
print(x)
Ví dụ sắp xếp giảm dần của trường name
import mysql.connector
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="mydatabase"
)
mycursor = mydb.cursor()
sql = "SELECT * FROM customers ORDER BY name DESC"
mycursor.execute(sql)
myresult = mycursor.fetchall()
for x in myresult:
print(x)
Giới hạn số lượng bản ghi trả về khi truy vấn dữ liệu
Khi bảng chứa rất nhiều bản ghi, do đó khi truy vấn dữ liệu trả về có thể rất nhiều bản ghi thỏa mãn, khi đó chúng ta sẽ giới hạn số lượng bản ghi nhất định bằng từ khóa LIMIT trong SQL. Ví dụ chọn 5 bản ghi đầu tiên thỏa mãn điều kiện của câu lệnh truy vấn.
import mysql.connector
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="mydatabase"
)
mycursor = mydb.cursor()
mycursor.execute("SELECT * FROM customers LIMIT 5")
myresult = mycursor.fetchall()
for x in myresult:
print(x)
Để lấy các bản ghi thỏa mãn điều kiện tại các vị trí bất kỳ ta sử dụng từ khóa OFFSET. Ví dụ để lấy 5 bản ghi bắt đầu từ vị trí thứ 3, dãy câu lệnh sẽ như sau:
import mysql.connector
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="mydatabase"
)
mycursor = mydb.cursor()
mycursor.execute("SELECT * FROM customers LIMIT 5 OFFSET 2")
myresult = mycursor.fetchall()
for x in myresult:
print(x)