10 bài tập cơ bản học lập trình C#
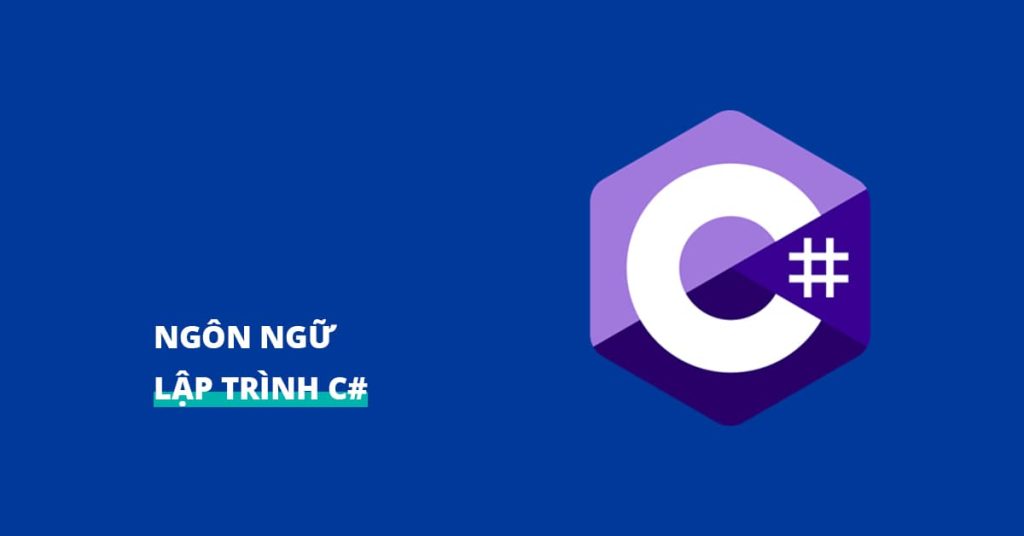
1. Tính tổng 2 số nhập vào từ bàn phím:
using System;
namespace Exercise1
{
class Program
{
static void Main(string[] args)
{
int num1, num2, sum;
Console.Write("Enter the first number: ");
num1 = int.Parse(Console.ReadLine());
Console.Write("Enter the second number: ");
num2 = int.Parse(Console.ReadLine());
sum = num1 + num2;
Console.WriteLine("The sum of {0} and {1} is {2}", num1, num2, sum);
Console.ReadLine();
}
}
}
2. Tính diện tích hình tròn:
using System;
namespace Exercise2
{
class Program
{
static void Main(string[] args)
{
double radius, area;
Console.Write("Enter the radius: ");
radius = double.Parse(Console.ReadLine());
area = Math.PI * radius * radius;
Console.WriteLine("The area of the circle is {0}", area);
Console.ReadLine();
}
}
}
3. Tìm số lớn nhất trong 3 số:
using System;
namespace Exercise3
{
class Program
{
static void Main(string[] args)
{
int num1, num2, num3;
Console.Write("Enter the first number: ");
num1 = int.Parse(Console.ReadLine());
Console.Write("Enter the second number: ");
num2 = int.Parse(Console.ReadLine());
Console.Write("Enter the third number: ");
num3 = int.Parse(Console.ReadLine());
int max = num1;
if (num2 > max)
{
max = num2;
}
if (num3 > max)
{
max = num3;
}
Console.WriteLine("The maximum number is {0}", max);
Console.ReadLine();
}
}
}
4. Tìm số nguyên tố:
using System;
namespace Exercise4
{
class Program
{
static void Main(string[] args)
{
int num;
Console.Write("Enter a number: ");
num = int.Parse(Console.ReadLine());
bool isPrime = true;
for (int i = 2; i <= num / 2; i++)
{
if (num % i == 0)
{
isPrime = false;
break;
}
}
if (isPrime)
{
Console.WriteLine("{0} is a prime number", num);
}
else
{
Console.WriteLine("{0} is not a prime number", num);
}
Console.ReadLine();
}
}
}
5. Tìm nghiệm của phương trình bậc 2:
using System;
namespace Exercise5
{
class Program
{
static void Main(string[] args)
{
double a, b, c, delta, x1, x2;
Console.Write("Enter the value of a: ");
a = double.Parse(Console.ReadLine());
Console.Write("Enter the value of b: ");
b = double.Parse(Console.ReadLine());
Console.Write("Enter the value of c: ");
c = double.Parse(Console.ReadLine());
delta = b * b - 4 * a * c;
if (delta < 0){
Console.WriteLine("No real roots");
}
else if (delta == 0) {
x1 = -b / (2 * a);
Console.WriteLine("The only root is {0}", x1);
}
else {
x1 = (-b + Math.Sqrt(delta)) / (2 * a);
x2 = (-b - Math.Sqrt(delta)) / (2 * a);
Console.WriteLine("The roots are {0} and {1}", x1, x2);
}
Console.ReadLine();
}
}
}
6. Tính tổng các số trong một mảng:
using System;
namespace MyProgram
{
class Program
{
static void Main(string[] args)
{
int[] arr = { 1, 2, 3, 4, 5 };
int sum = 0;
for (int i = 0; i < arr.Length; i++)
{
sum += arr[i];
}
Console.WriteLine("Total number is: " + sum);
}
}
}
7. Kiểm tra một chuỗi có phải là palindrome không:
using System;
namespace Exercise7
{
class Program
{
static void Main(string[] args)
{
string str, reverse = "";
Console.Write("Enter a string: ");
str = Console.ReadLine();
for (int i = str.Length - 1; i >= 0; i--)
{
reverse += str[i];
}
if (str == reverse)
{
Console.WriteLine("{0} is a palindrome", str);
}
else
{
Console.WriteLine("{0} is not a palindrome", str);
}
Console.ReadLine();
}
}
}
8. In bảng cửu chương:
using System;
namespace Exercise8
{
class Program
{
static void Main(string[] args)
{
for (int i = 1; i <= 9; i++)
{
for (int j = 1; j <= 9; j++)
{
Console.Write("{0}x{1}={2}\t", j, i, i * j);
}
Console.WriteLine();
}
Console.ReadLine();
}
}
}
9. Tìm các số chia hết cho 3 hoặc 5 trong khoảng từ 1 đến 100:
using System;
namespace Exercise9
{
class Program
{
static void Main(string[] args)
{
for (int i = 1; i <= 100; i++)
{
if (i % 3 == 0 || i % 5 == 0)
{
Console.WriteLine(i);
}
}
Console.ReadLine();
}
}
}
10. Tính số Fibonacci thứ n:
using System;
namespace Exercise10
{
class Program
{
static void Main(string[] args)
{
int n, n1 = 0, n2 = 1, n3;
Console.Write("Enter the value of n: ");
n = int.Parse(Console.ReadLine());
if (n == 1)
{
Console.WriteLine("The {0}st Fibonacci number is 0", n);
}
else if (n == 2)
{
Console.WriteLine("The {0}nd Fibonacci number is 1", n);
}
else if (n == 3)
{
Console.WriteLine("The {0}rd Fibonacci number is 1", n);
}
else
{
for (int i = 3; i <= n; i++)
{
n3 = n1 + n2;
n1 = n2;
n2 = n3;
}
Console.WriteLine("The {0}th Fibonacci number is {1}", n, n2);
}
Console.ReadLine();
}
}
}
Trên đây là 10 bài tập lập trình C# cơ bản để các bạn luyện tập ngôn ngữ lập trình C#.